PowerShell | Script to migrate a server from on-premises to the cloud
- Aakash Rahsi
- Aug 23, 2024
- 3 min read
Updated: Sep 16, 2024
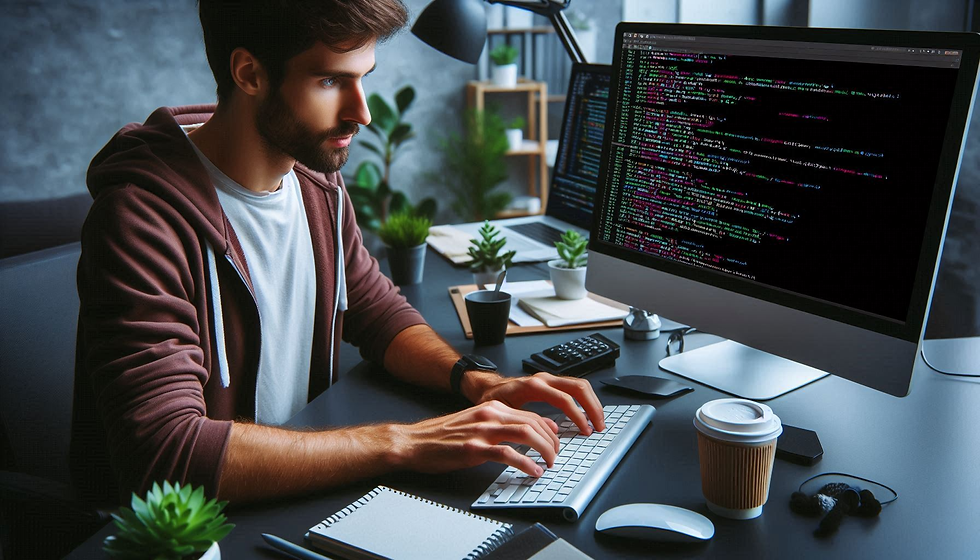
Prerequisites:
Azure PowerShell Module: Ensure the installation of the Azure PowerShell Module. If not, you can download and install it from here:
powershell
Install-Module -Name Az -AllowClobber -Force
Azure Subscription: Make sure you have an active Azure subscription and the appropriate rights.
On-premises VM: Ensure that the on-premises VM is ready to migrate.
PowerShell Script:
powershell
Step 1: Connect to your Azure account
# This will open a window asking you for your Azure credentials.
Connect-AzAccount
Step 2: Select the Azure Subscription you would like to work with
# If you have multiple subscriptions, specify which one you'd like to work with.
$subscriptionId = "your-subscription-id"
Select-AzSubscription -SubscriptionId $subscriptionId
Step 3: Define variables for the migration
# These host the VM, Storage, and resource group information.
$resourceGroupName = "your-resource-group-name"
$location = "EastUS" # Pick your Azure region closest to your users
$vmName = "your-vm-name" # Name of the on-prem VM
$vhdUri = "https://yourstorageaccount.blob.core.windows.net/vhds/your-vm.vhd" # VHD file path in Azure Storage
$vmSize = "Standard_DS1_v2" # VM size in Azure
Step 4: Create a resource group in Azure (if not already existing)
# Resource groups in Azure are containers that hold related resources.
if (-Not (Get-AzResourceGroup -Name $resourceGroupName -ErrorAction SilentlyContinue)) {
New-AzResourceGroup -Name $resourceGroupName -Location $location
}
Step 5: Create a storage account in Azure (if not already existing)
# This storage account will hold the VM's VHD file.
$storageAccountName = "yourstorageaccount"
if(-Not(Get-AzStorageAccount -ResourceGroupName $resourceGroupName -Name $storageAccountName -ErrorAction SilentlyContinue)) {
$storageAccount = New-AzStorageAccount -ResourceGroupName $resourceGroupName `
-Name $storageAccountName -Location $location -SkuName "Standard_LRS"
} else {
$storageAccount = Get-AzStorageAccount -ResourceGroupName $resourceGroupName -Name $storageAccountName
}
Step 6: Upload the VHD file to Azure Blob Storage
$storageContext = $storageAccount.Context
$vhdBlobName = "your-vm.vhd"
$vhdBlobUri = "https://$($storageAccountName).blob.core.windows.net/vhds/$vhdBlobName"
Set-AzStorageBlobContent -File "C:\path\to\your-vm.vhd" -Container "vhds" -Blob $vhdBlobName -Context $storageContext
Step 7: Create a virtual network and subnet (if not already existing)
# The VM will be deployed into this virtual network.
$vnetName = "your-vnet-name"
$subnetName = "your-subnet-name"
$vnet = Get-AzVirtualNetwork -ResourceGroupName $resourceGroupName -Name $vnetName -ErrorAction SilentlyContinue
if (-Not $vnet) {
$subnetConfig = New-AzVirtualNetworkSubnetConfig -Name $subnetName -AddressPrefix "10.0.0.0/24"
$vnet = New-AzVirtualNetwork -ResourceGroupName $resourceGroupName -Location $location `
-Name $vnetName -AddressPrefix "10.0.0.0/16" -Subnet $subnetConfig
} else {
$subnetConfig = $vnet.Subnets | Where-Object { $_.Name -eq $subnetName }
}
Step 8: Create a public IP address for the VM
$publicIpName = "$vmName-pip"
$publicIp = New-AzPublicIpAddress -ResourceGroupName $resourceGroupName -Location $location `
-Name $publicIpName -AllocationMethod Dynamic
Step 9: Create a network interface for the VM
# A network interface is associated with a VM and virtual network.
$nicName = "$vmName-nic"
$nic = New-AzNetworkInterface -Name $nicName -ResourceGroupName $resourceGroupName -Location $location `
-SubnetId $subnetConfig.Id -PublicIpAddressId $publicIp.Id
Step 10: Now create the configuration for the virtual machine which will define the OS type, VM size, and other parameters.
$vmConfig = New-AzVMConfig -VMName $vmName -VMSize $vmSize | `
Set-AzVMOperatingSystem -Windows -ComputerName $vmName -ProvisionVMAgent -EnableAutoUpdate | `
Set-AzVMSourceImage -VhdUri $vhdBlobUri | `
Set-AzVMOSDisk -VhdUri $vhdBlobUri -CreateOption FromImage -OSState Generalized -OSType Windows | `
Add-AzVMNetworkInterface -Id $nic.Id
Step 11: Create the VM in Azure
New-AzVM -ResourceGroupName $resourceGroupName -Location $location -VM $vmConfig
Step 12: Cleanup (Optional)
# Optionally, you can remove the on-premises VM after confirming the migration.
# Remove-VM -Name $vmName -Force
Write-Host "Migration completed successfully."
Explanation:
Step 1: Connects to your Azure account.
Step 2: Selects the Azure subscription you want to use.
Step 3: Defines variables for the migration, including resource group name, location, VM name, etc.
Step 4: Checks if the resource group exists; otherwise, create one.
Step 5: Checks if a storage account exists; otherwise, create one.
Step 6: Uploads the VHD file to Azure Blob Storage.
Step 7: Creates a virtual network and subnet if they don’t exist.
Step 8: Creates a public IP address for external access.
Step 9: Creates a NIC for this VM.
Step 10: Configures the VM with required settings.
Step 11: Deploys an Azure VM based on the configuration.
Step 12: (Optional) Deleting on-premises VM once migration has been verified to be working satisfactorily.
Comments