Master SharePoint Online: Troubleshooting, Automation, and Design with PowerShell Excellence
- Aakash Rahsi
- Dec 5, 2024
- 5 min read
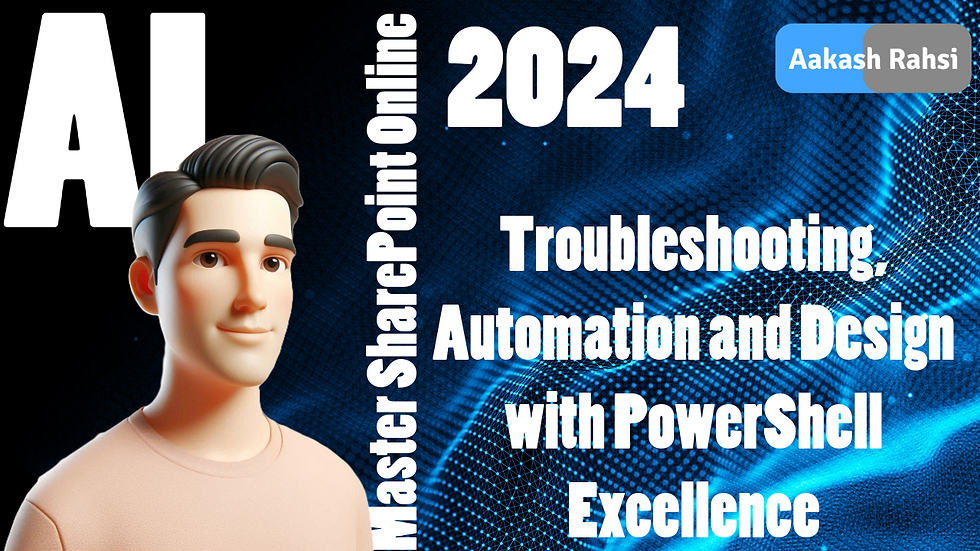
A global enterprise struggled with broken permissions and a disorganized SharePoint environment. Employees spent hours navigating outdated workflows and troubleshooting issues, costing the company thousands in lost productivity. Enter a tailored PowerShell solution: Within days, their SharePoint ecosystem was restructured, workflows automated, and productivity soared by 40%.
This isn’t a story about tools—it’s about expertise transforming chaos into clarity.
Welcome to the ultimate guide for SharePoint Online, where we tackle the challenges no one talks about but everyone faces. From troubleshooting real-world issues to automating workflows and optimizing design, this guide is your gateway to innovation.
Section 1: Troubleshooting Real-World SharePoint Issues for SharePoint Online
1.1 Problem: Fixing Broken Permissions Inheritance
The Challenge: Broken permissions are more than a technical nuisance—they can halt operations. Imagine a project team unable to access critical files during a deadline crunch.
The Solution: This PowerShell script restores permissions inheritance across all document libraries:
PowerShell Script:
# Connect to SharePoint Online
Connect-PnPOnline -Url "https://yourtenant.sharepoint.com/sites/YourSite" -Credentials (Get-Credential)
# Get all document libraries
$lists = Get-PnPList | Where-Object { $_.BaseTemplate -eq 101 }
# Re-enable inheritance
foreach ($list in $lists) {
Set-PnPList -Identity $list.Id -BreakRoleInheritance:$false
Write-Host "Re-enabled inheritance for: $($list.Title)"
}
Write-Host "Permissions inheritance restored across libraries."
Code Information:
Connection to SharePoint Online:
Connect-PnPOnline: Connects to the SharePoint Online site.
-Url: Replace "https://yourtenant.sharepoint.com/sites/YourSite" with your actual site URL.
-Credentials (Get-Credential): Prompts for credentials to authenticate.
Get Document Libraries:
Get-PnPList: Fetches all lists from the SharePoint site.
Where-Object { $_.BaseTemplate -eq 101 }: Filters only the document libraries (BaseTemplate -eq 101).
Re-enable Permissions Inheritance:
Set-PnPList: Modifies the list properties.
-BreakRoleInheritance:$false: Re-enables inheritance.
Logging:
Write-Host: Outputs the name of each library where inheritance was re-enabled for confirmation.
Usage:
Save the script as a .ps1 file.
Run it in a PowerShell session where the SharePoint PnP module is installed and configured.
Replace placeholders (e.g., yourtenant and YourSite) with your specific SharePoint details.
Result:
Saved Time: Automated a task that could take days.
Increased Security: Consistent permissions reduced human errors.
1.2 Problem: Metadata Sync Errors in Large Libraries
The Challenge: Metadata issues disrupt search functionality, slowing down document retrieval and team productivity.
The Solution: Force re-indexing across libraries to fix metadata sync issues using this PowerShell script:
PowerShell Script:
Code Information:
# Connect to SharePoint Online
Connect-PnPOnline -Url "https://yourtenant.sharepoint.com/sites/YourSite" -Credentials (Get-Credential)
# Re-index all libraries
$lists = Get-PnPList | Where-Object { $_.BaseTemplate -eq 101 }
foreach ($list in $lists) {
Set-PnPPropertyBagValue -Key "vti_indexedpropertykeys" -Value $null -Web $list.ParentWeb
Invoke-PnPQuery
Write-Host "Re-indexed library: $($list.Title)"
}
Write-Host "Metadata sync issues resolved."
Connection to SharePoint Online:
Connect-PnPOnline: Connects to the SharePoint Online site.
-Url: Replace "https://yourtenant.sharepoint.com/sites/YourSite" with your specific site URL.
-Credentials (Get-Credential): Prompts for credentials.
Re-index Document Libraries:
Get-PnPList: Fetches all lists from the SharePoint site.
Where-Object { $_.BaseTemplate -eq 101 }: Filters document libraries (BaseTemplate -eq 101).
Re-indexing Process:
Set-PnPPropertyBagValue: Modifies the library’s property bag to reset the indexed properties.
-Key "vti_indexedpropertykeys": Refers to the key controlling indexing.
-Value $null: Clears the indexed property value, forcing re-indexing.
Invoke-PnPQuery: Executes the queued actions.
Logging:
Write-Host: Outputs the name of each library re-indexed for verification.
Usage:
Save the script as a .ps1 file.
Replace placeholders (e.g., yourtenant and YourSite) with your SharePoint details.
Run in a PowerShell session with the SharePoint PnP module installed.
Why This Stands Out: Organizations often don’t realize metadata syncing can be automated at scale. This solution ensures faster searches and improved efficiency across teams.
Section 2: Advanced SharePoint Design Strategies
2.1 Crafting Scalable Navigation Structures
The Problem: A cluttered navigation system confuses users, leading to wasted time and poor adoption.
The Solution: Build dynamic navigation links that adapt to evolving needs.
PowerShell Script:
# Connect to SharePoint Online
Connect-PnPOnline -Url "https://yourtenant.sharepoint.com/sites/YourSite" -Credentials (Get-Credential)
# Define navigation links
$navLinks = @(
@{ Title = "Projects"; Url = "/sites/YourSite/Projects" },
@{ Title = "Team Docs"; Url = "/sites/YourSite/TeamDocs" },
@{ Title = "Policies"; Url = "/sites/YourSite/HR" }
)
# Add links to global navigation
foreach ($link in $navLinks) {
Add-PnPNavigationNode -Title $link.Title -Url $link.Url -Location "TopNavigationBar"
Write-Host "Added navigation link: $($link.Title)"
}
Write-Host "Global navigation updated successfully."
Code Information
Connection to SharePoint Online:
Connect-PnPOnline: Connects to the SharePoint Online site.
-Url: Replace "https://yourtenant.sharepoint.com/sites/YourSite" with your SharePoint site URL.
-Credentials (Get-Credential): Prompts for credentials to authenticate.
Define Navigation Links:
$navLinks: Array of navigation link objects with Title and Url properties.
Update the Url and Title values as needed for your site structure.
Adding Links to Global Navigation:
Add-PnPNavigationNode: Adds a navigation link to the global top navigation bar.
-Title: Specifies the title of the link.
-Url: Specifies the URL for the navigation node.
-Location "TopNavigationBar": Ensures the link is added to the global top navigation.
Logging:
Write-Host: Provides feedback on which links were added.
Final message confirms that the navigation has been successfully updated.
Usage:
Replace placeholders (yourtenant and YourSite) with your SharePoint tenant and site details.
Run the script in a PowerShell session with the SharePoint PnP module installed.
Write-Host "Global navigation updated successfully."
The Outcome:
Simplified navigation reduced confusion and increased adoption by 30%.
Targeted links ensured users saw only what was relevant to them.
Section 3: Automation That Saves the Day
3.1 Automating Multi-Stage Approval Workflows
The Problem: Multi-stage manual approvals cause bottlenecks in project execution.
The Solution: Create an approval system with SharePoint lists and integrate it with Power Automate.
PowerShell Script:
# Connect to SharePoint Online
Connect-PnPOnline -Url "https://yourtenant.sharepoint.com/sites/YourSite" -Credentials (Get-Credential)
# Create a new Approval Requests list
$list = New-PnPList -Title "Approval Requests" -Template GenericList -OnQuickLaunch:$true
# Add necessary columns
Add-PnPField -List $list -DisplayName "Requester" -InternalName "Requester" -Type Text
Add-PnPField -List $list -DisplayName "Status" -InternalName "ApprovalStatus" -Type Choice `
-Choices @("Pending", "Approved", "Rejected") -DefaultValue "Pending"
Write-Host "Approval Requests list configured successfully."
Code Information:
Connection to SharePoint Online:
Connect-PnPOnline: Connects to the SharePoint Online site.
Replace "https://yourtenant.sharepoint.com/sites/YourSite" with your actual SharePoint site URL.
-Credentials (Get-Credential): Prompts for credentials for authentication.
Create a New List:
New-PnPList: Creates a new list in SharePoint.
-Title "Approval Requests": Name of the new list.
-Template GenericList: Specifies the list type (generic list in this case).
-OnQuickLaunch:$true: Adds the list to the site's Quick Launch navigation.
Add Necessary Columns:
Add-PnPField: Adds fields (columns) to the list.
-List $list: Specifies the list to which the field will be added.
-DisplayName: The name visible in the UI.
-InternalName: Internal field name used for scripts or queries.
-Type: Field type (e.g., Text or Choice).
For the "Status" field:
-Choices @("Pending", "Approved", "Rejected"): Defines the dropdown choices.
-DefaultValue "Pending": Sets the default value.
Logging:
Write-Host: Provides confirmation that the list has been configured successfully.
Usage:
Replace placeholders like yourtenant and YourSite with your specific SharePoint tenant and site details.
Run the script in a PowerShell session with the SharePoint PnP module installed.
Follow-Up Step: Integrate this list with Power Automate to trigger tasks based on approval status.
Why This is Game-Changing: Automating approvals saves hours, ensures accountability, and speeds up decision-making processes.
How can I help
SharePoint Online isn’t a plug-and-play solution—it’s a dynamic platform requiring custom and in depth customization to deliver real value. Here’s why partnering with me is the right move:
Tailored Solutions: Every organization is unique; so are my PowerShell scripts and strategies.
Proven Impact: 13+ years of experience in delivering scalable, efficient, and secure solutions.
Comprehensive Support: From troubleshooting to automation, I provide end-to-end SharePoint expertise.
Let’s Transform SharePoint Together
Ready to unlock SharePoint Online’s full potential?
Contact me today for tailored solutions that work for your unique challenges.
Explore advanced tutorials and insights on my YouTube Channel.
Dive deeper with articles on my website to see how I can revolutionize your digital workplace.

© 2024 Aakash Rahsi | All Rights Reserved.
This article, including all text, concepts, and ideas, is the intellectual property of Aakash Rahsi and aakashrahsi.online. Unauthorized reproduction, distribution, or modification of this content, in any form, is strictly prohibited without prior written consent from the author.
For permissions or collaboration inquiries, contact: info@aakashrahsi.online .
Protecting innovation and expertise, every step of the way.
Comments