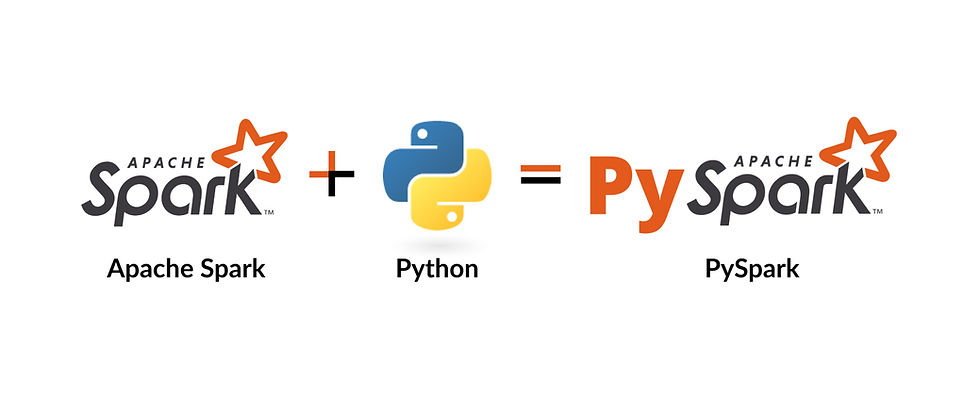
What is PySpark?
PySpark is the Python API for Apache Spark, a distributed computing system. It allows users to write Spark applications using Python, facilitating seamless integration with existing Python libraries and frameworks. PySpark is particularly valuable for handling big data, as it can efficiently process large datasets across a cluster of computers.
Why Use PySpark?
There are several reasons to consider using PySpark:
Speed: PySpark can process data up to 100 times faster than traditional MapReduce.
Ease of Use: It offers high-level APIs in Python, making it accessible to developers familiar with Python.
Compatibility: PySpark runs on various platforms, including Hadoop, Kubernetes, and cloud services like AWS and Azure.
Machine Learning Support: PySpark includes MLlib, a scalable machine learning library.
DataFrame API: Similar to pandas, the DataFrame API allows easy data manipulation.
Installing PySpark
Before starting with PySpark, you need to install it. Here’s a straightforward way to get started:
Ensure Python is installed on your system.
Open your terminal or command prompt.
Create a virtual environment (recommended) using python -m venv myenv.
Activate the virtual environment:
Windows: myenv\Scripts\activate
macOS/Linux: source myenv/bin/activate
Install PySpark using pip: pip install pyspark.
To verify the installation, run import pyspark in your Python environment.
Getting Started with PySpark
To begin using PySpark, you need to create a Spark session, which is the entry point for using PySpark functionalities.
python
from pyspark.sql import SparkSession spark = SparkSession.builder \ .appName("My PySpark Application") \ .getOrCreate()
This code initializes a Spark session named "My PySpark Application." Once the session is created, you can start leveraging various PySpark functionalities.
Working with DataFrames
DataFrames are a key feature of PySpark, allowing for easy manipulation of structured data. Here’s how to create a DataFrame:
python
data = [("Alice", 1), ("Bob", 2), ("Cathy", 3)] columns = ["Name", "Id"] df = spark.createDataFrame(data, schema=columns) df.show()
This code creates a DataFrame with two columns: Name and Id. The show() method displays the DataFrame’s content.
Reading Data from CSV Files
PySpark simplifies reading data from various sources, including CSV files. Here’s how to read a CSV file into a DataFrame:
python
df = spark.read.csv("path/to/your/file.csv", header=True, inferSchema=True) df.show()
Here, header=True indicates that the first row contains column names, and inferSchema=True enables PySpark to automatically detect each column’s data type.
Handling Missing Values
Data often contains missing values, which can pose challenges for analysis. PySpark offers methods to handle missing data:
Data Aggregation and Grouping
PySpark also excels at performing data aggregation and grouping. Here’s an example of grouping data and calculating aggregates:
python
df.groupBy("column_name").agg({"another_column": "sum"}).show()
This code groups the DataFrame by a specified column and calculates the sum of another column.
Introduction to MLlib
MLlib is PySpark’s scalable machine learning library, offering various algorithms for tasks like classification, regression, and clustering. To use MLlib, you typically need to prepare your data by transforming it into a feature vector.
python
from pyspark.ml.feature import VectorAssembler assembler = VectorAssembler(inputCols=["feature1", "feature2"], outputCol="features") transformed_data = assembler.transform(df) transformed_data.show()
This code creates a new column named "features" that combines the specified input columns into a single feature vector.
Implementing Linear Regression
Once your data is prepared, you can implement machine learning algorithms such as linear regression:
python
from pyspark.ml.regression import LinearRegression lr = LinearRegression(featuresCol="features", labelCol="label_column") model = lr.fit(transformed_data)
This code fits a linear regression model to your data, allowing you to evaluate the model’s performance using various metrics.
Using Databricks for PySpark
Databricks is a cloud-based platform optimized for running Apache Spark. It offers features like collaborative notebooks, easy cluster management, and built-in visualization tools. You can run your PySpark code in Databricks by creating a new notebook and executing your code in cells.
Conclusion
PySpark is a powerful tool for processing large datasets and implementing machine learning algorithms. Its ability to handle big data efficiently makes it an essential skill for data scientists and engineers. This guide has provided you with a solid foundation for getting started with PySpark, manipulating data using DataFrames, and implementing machine learning models.
Comments